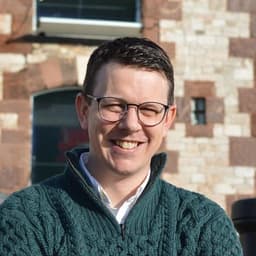
by Dr Seán Carroll
Python 101 - The (very) Basics
15. Control flow
The next big element we need to cover is control flow or conditional programming. This is the ability for our code to 'make decisions' about what code to run based on conditions that we specify. This is achieved by using three keywords: if
, elif
and else
. With this tool we can encode a huge range of behaviours into our code.
The basic structure of a conditional statement in Python is as follows:
if condition1:
# code block to execute if condition1 is True
elif condition2:
# code block to execute if condition1 is False and condition2 is True
else:
# code block to execute if both condition1 and condition2 are False
The if
statement is the most basic form of conditional statement. It is followed by a condition, which is a boolean expression. If the condition is True
then the code block that follows is executed. If the condition is False
then the code block is skipped and the next line of code is executed.
The elif
statement is an optional extension to the if
statement that behaves in the same way. It's followed by a condition, which is a boolean expression. If the condition is True
then the code block that follows is executed. If the condition is False
then the code block is skipped.
The else
statement is a further optional extension to the if
statement. It is followed by a code block that executes if none of the preceding conditions are True
. It does not have a condition associated with it.
Booleans and comparison operators
Before we can use conditional statements we need to understand what a boolean is and how to make comparisons between variables using comparison operators. A boolean is another fundamental data type in Python (and other languages) used to represent the truth values: True
and False
. Booleans are used extensively in conditional statements, loops, and comparisons to help make decisions and control the flow of a programme.
We can create boolean values by directly assigning True
or False
(note the capital letters) to a variable or by using a comparison operator or boolean function.
For example, we can create a new variable called my_bool
and assign it the value True
as follows:
my_bool = True
print(f"my_bool has a value of {my_bool}")
# Output: 'my_bool has a value of True'
Booleans really become useful as a means of holding the result of a comparison between two variables. Put simply, we may have two variables, a
and b
and we may want to compare them to determine if a
is greater than b
. The result of this comparison is a boolean value, either True
or False
. So, if booleans hold the result of a comparison, the next thing we need to do is work out how to make the comparison. We do this with comparison operators and there are 6 different types.
Equality operator
The equality operator (==)
checks if two values are equal. It returns True
if they are and False
otherwise. For example:
#Define some variables
x = 10
y = 20
#Define a boolean, 'result', as the output of the equality operator applied to x and y
result = x == y
print(f"result has a value of {result}")
# Output: 'result has a value of False'
Another way to think about this is that the equality operator is a function that takes two arguments and returns a boolean. The arguments are the two variables that are being compared. The boolean is the output of the function. The function is called '=='.
We can get another more intuitive demonstration by using the if
keyword we saw earlier. We can use the equality operator to check if two variables are equal and then execute a code block if they are. For example:
x=10
y=20
if x==y:
print("x is equal to y")
else:
print("x is not equal to y")
# Output: 'x is not equal to y'
Inequality operator
The inequality operator (!=)
checks if two values are not equal and returns True
if they are and False
otherwise. It operates just like the equality operator but returns the opposite result. For example:
x=10
y=20
if x!=y:
print("x is not equal to y")
else:
print("x is equal to y")
# Output: 'x is not equal to y'
Greater than operator
The greater than operator (>)
checks if the left operand is greater than the right operand and returns True
if it is and False
if not:
x=30
y=20
if x>y:
print("x is greater than y")
else:
print("x is not greater than y")
# Output: 'x is greater than y'
Less than operator
The less than operator (<)
is simply the reverse of the greater than operator. It checks if the left operand is less than the right operand, returning True
if it is and False
if it's not:
x=30
y=20
if x<y:
print("x is less than y")
else:
print("x is not less than y")
# Output: 'x is not less than y'
Greater than or equal to operator
The greater than or equal to operator (>=)
checks if the left operand is greater than or equal to the right operand. It returns True
if it is and False
otherwise:
x=30
y=20
if x>=y:
print("x is greter than or equal to y")
else:
print("x is not greater than or equal to y")
# Output: 'x is greater than or equal to y'
Less than or equal to operator
Finally, we have the less than or equal to operator (<=)
which checks if the left operand is less than or equal to the right operand. It returns True
if it is and False
otherwise:
x=30
y=20
if x<=y:
print("x is less than or equal to y")
else:
print("x is not less than or equal to y")
# Output: 'x is not less than or equal to y'
Combining comparisons
We can also use the and
and or
operators to combine multiple comparisons. This allows us to build up more complex conditions. For example, we can check if a variable is greater than 10 and less than 20 as follows:
x=15
if x > 10 and x < 20:
print("x is greater than 10 and less than 20")
# Output: 'x is greater than 10 and less than 20'
The or
operator is used to check if either of two conditions are True
. For example, we can check if a variable is less than 10 or greater than 20 as follows:
x=5
if x < 10 or x > 20:
print("x is outside the range 10 to 20")
# Output: 'x is outside the range 10 to 20'
Returning to break
and continue
Recall in the last lesson we said that break
and continue
are used to control the flow of a loop. Well, now that we understand conditional statements, we can see how to use break
and continue
in a loop.
break
The break
keyword is used to exit a loop. For example, we can use it to exit a loop if a condition is met. For example:
for i in range(10):
if i == 5:
break
print(i)
The output is,
0
1
2
3
4
Here, we've used the conditional if statement to check if the current value of i
is equal to 5. If it is, we use the break
keyword to exit the loop. If it's not, we print the value of i
and continue to the next iteration of the loop.
Continue
The continue
keyword is used to skip the current iteration of a loop and continue to the next iteration. For example:
for i in range(10):
if i == 5:
continue
print(i)
This yields the output,
0
1
2
3
4
6
7
8
9
Exercise
Everything we've covered so far in this section has been relatively simple. We've covered the basics of variables, data types, function definitions, loops and conditional statements. It's not the most exhilerating stuff but it's the foundation of all programming and believe it or not it equips us with the tools to do perform some pretty elaborate data analysis and processing. We have essentially established a toolbox of tools that allows us to capture logic and decision making into our code.
Now it's time to try and put what we've learned so far into practice. Consider the problem statement below and try and write a programme that solves it. I'll come back in the next lesson and walk you through my solution Make sure to pause here and try and solve the problem before moving on - you'll learn far more than if you just watch me walk through the solution.
Problem statement
A car rental company needs a programme to calculate the pricing of its car rentals based on the following criteria:
-
The base price per day: $50
-
Additional fees:
- For each 100 miles driven: $20
- For each day the car is rented on the weekend (Saturday or Sunday): $10
- Discounts:
- For rentals lasting 7 or more days: 15% off the total price
- For rentals with more than 500 miles driven: 10% off the total price
The company wants the programme to take the following inputs:
- The number of days the car is rented.
- The total miles driven during the rental period.
- The start day of the rental (Monday, Tuesday, Wednesday, etc.).
The script should calculate the total price of the rental, applying any applicable additional fees and discounts, and output the final price.
Right - go put the kettle on and give it a go. I'll be back in the next lesson to walk you through my solution.