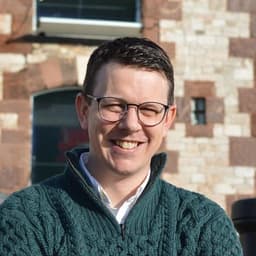
by Dr Seán Carroll
Python 101 - The (very) Basics
14. Loops in Python
Now let's talk about loops. Loops are a fundamental concept in programming that makes it possible to execute a block of code multiple times as long as a specific condition is met. This can greatly improve the efficiency of your code. They're particularly helpful when you need to perform repetitive tasks, such as iterating over a sequence of values, running calculations on a set of data points, or processing user input.
Python has two main types of loops: for
loops and while
loops. Both types can be used to execute a block of code multiple times, but they differ in how they determine the number of repetitions. We'll start by considering for
loops.
The for
loop
The for
loop is used to iterate over a sequence (such as a list, tuple, string, or dictionary) and execute a block of code for each item in the sequence. The general syntax for a for loop is as follows:
numbers = [1, 2, 3, 4, 5] # Some list of values we want to iterate over
for num in numbers:
# some code to execute for each value in the list
print(num)
This code will print each number in the list on a separate line...
1
2
3
4
5
Admittedly, this is a fairly trivial example, but it illustrates the basic structure of a for
loop. The for
keyword is followed by a variable name, which is used to refer to each item in the sequence. The in
keyword is followed by the sequence itself. The colon at the end of the line indicates the start of the loop’s body.
The body of the for
loop is indented (just like when we defined a function), and the indentation is used to indicate which statements are part of the loop. The body of the loop is executed once for each item in the sequence. The variable name is assigned the value of the next item in the sequence each time the loop executes. When there are no more items in the sequence, the loop terminates.
Very often we will need to iterate over the items in a collection and perform some operation on each item. Consider our user
dictionary from a couple of lessons ago:
user = {'name': 'John', 'age': 25, 'hobbies': ['Playing Music', 'Watching Movies']}
print(user)
{'name': 'John', 'age': 25, 'hobbies': ['Playing Music', 'Watching Movies']}
We could imagine a scenario where we might need to iterate over the different parts of the user's profile and do something with the data. In the simplest case, we might just print them out. We could do this using a for
loop as follows:
for key in user:
print(f"{key}: {user[key]}")
Yielding,
name: John
age: 25
hobbies: ['Playing Music', 'Watching Movies']
It's also very common to want to generate a counter variable that keeps track of the number of times the loop has executed. This is particularly useful when we want to iterate over a collection that is not itself just a sequence of numbers - like the case above.
We can add a counter variable that increments by one with every loop iteration using the enumerate()
function. Here's a simple example:
for index, key in enumerate(user, start=0):
# code to be executed
print(f"{index} - {key}: {user[key]}")
Yielding,
0 - name: John
1 - age: 25
2 - hobbies: ['Playing Music', 'Watching Movies']
Here, user
is again the iterable we want to loop over, index
represents the position of the current item in the iterable, and key
is the item itself. The optional start parameter specifies the starting value of the index (the default value is 0...for programmers, but normal people might like to start at 1!)
The while
loop
The while
loop in Python executes a block of code as long as a specific condition is true. Let's consider an example:
count = 1 # initialise the counter
while count <= 5:
print(count)
count += 1 # increment the counter on each iteration
This results in the following output:
1
2
3
4
5
While loops are useful when we don’t know how many times we want to execute a block of code in advance. For example, we might want to keep iterating on a calculation until we reach a certain level of accuracy or until a certain condition is met. Here’s an example of a while loop that iterates until a threshold value is reached.
Let’s imagine we have two numbers and we want to successively half the difference between them until the difference is less than 1% of the original difference. Technically we could work out the number of iterations this would take and run a for loop with this pre-defined number of iterations. But it’s probably easier to let a while loop continue running until the condition is met. So that we can package up this code for easy re-use, let’s define a function that takes two numbers as input and returns the number of iterations required to reach the threshold:
def half_diff(x, y, threshold=0.01):
"""Calculate the number of iterations required to reach a threshold
value when successively halving the difference between two numbers.
Parameters
----------
x : float
First number
y : float
Second number
threshold : float, optional
Threshold value (default is 0.01)
Returns
-------
int:
Number of iterations required to reach the threshold value
"""
diff = abs(x - y) #Initial difference
count = 0 # Initialise the counter
while diff > threshold:
diff /= 2 #Same as diff = diff / 2
count += 1 #Same as count = count + 1
print(f"diff: {diff}, count: {count}")
return count
Now we can call this function with any two numbers and it will return the number of iterations required to reach the threshold.
half_diff(1, 2, 0.1)
Resulting in,
diff: 0.5, count: 1
diff: 0.25, count: 2
diff: 0.125, count: 3
diff: 0.0625, count: 4
4
Loop control statements
We can use the break
and continue
statements to change the normal flow of a loop. The break
statement is used to terminate a loop, and the continue
statement is used to skip the current iteration and continue with the next iteration.
Both of these features really become useful when combined with conditional statements that we’ll cover in the next lesson. So we’ll stick a pin in control statements for now and come back to them in the next lesson.